As Gutenberg evolves and becomes used for more than just creating blog posts, you may want the editor to look different depending on the type of content you’re editing.
For example, if you’re editing a blog post, you might want to set the editor width to 800px to contain your content so that it looks similar to your post on the front-end.
Or if you’re creating a landing page with a block plugin (Cwicly is my new favorite), you might want the editor to look more like a full-width blank canvas.
Beyond just modifying how your content is framed, you may also want to pull in external CSS stylesheets to apply to your content in Gutenberg.
For example, let’s say you’re using a page builder like Bricks Builder or Oxygen and have button styling applied to a .btn class.
Creating a button in Gutenberg with the class .btn won’t use that styling, as Gutenberg doesn’t pull in your external stylesheets.
Luckily, with a bit of code you’re able to specify which stylesheets you’d like to include in Gutenberg and where.
In this post, I’m going to show you how you can conditionally add Gutenberg editor styling based on post types, taxonomies and tags.
This will give you a lot more control – allowing you to make Gutenberg look exactly the way you want it.
Creating the PHP Snippet
We can add CSS stylesheets to Gutenberg using a simple code snippet.
You can either place this PHP snippet in your functions.php file or use a code snippet manager.
I prefer using code snippet manager approach since you maintain your snippets even if you happen to change themes in the future.
There are a few good code snippet manager plugins available for WordPress.
Code Snippets is a popular free option, but WPCodeBox is my favorite and easily worth the upgrade if you want full control over your snippets.
(WPCodeBox actually let’s you create external CSS files directly from the editor which is really cool. That means you don’t need to manually create & upload your CSS files).
Create a new PHP snippet, and call it something like “Add conditional Gutenberg styling.”
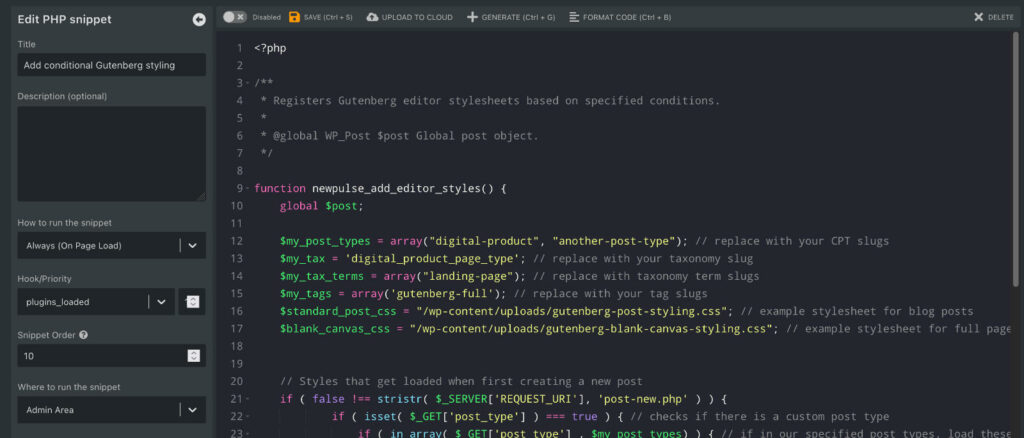
Since we’re only dealing with Gutenberg here, you can choose to only have it run in the Admin area.
Context for our example
Before I give you the snippet to copy/paste, I’ll briefly explain what’s going on in this example.
I am loading a gutenberg-post-styling.css stylesheet for blog posts, and a gutenberg-blank-canvas-styling.css stylesheet for landing pages.
How am I defining a landing page?
Well, that’s where our conditions come in.
Since I’m trying to provide you as many options as possible, I’m telling Gutenberg to load my blank canvas styling for posts that:
- are the “digital-product” custom post type
- or have the landing-page taxonomy term for my digital_product_page_type taxonomy
- or have the tag gutenberg-full tag
Here’s the full snippet:
<?php
/**
* Registers Gutenberg editor stylesheets based on specified conditions.
*
* @global WP_Post $post Global post object.
*/
function newpulse_add_editor_styles() {
global $post;
$my_post_types = array("digital-product", "another-post-type"); // replace with your CPT slugs
$my_tax = 'digital_product_page_type'; // replace with your taxonomy slug
$my_tax_terms = array("landing-page"); // replace with taxonomy term slugs
$my_tags = array("gutenberg-full"); // replace with your tag slugs
$standard_post_css = "https://newpulselabs.b-cdn.net/wp-content/uploads/gutenberg-post-styling.css"; // example stylesheet for blog posts
$blank_canvas_css = "/wp-content/uploads/gutenberg-blank-canvas-styling.css"; // example stylesheet for full pages
// Styles that get loaded when first creating a new post
if ( false !== stristr( $_SERVER['REQUEST_URI'], 'post-new.php' ) ) {
if ( isset( $_GET['post_type'] ) === true ) { // checks if there is a custom post type
if ( in_array( $_GET['post_type'] , $my_post_types) ) { // if in our specified post types, load these styles
add_editor_style( get_site_url() . $blank_canvas_css . '?v=' . filemtime( ABSPATH . $blank_canvas_css ) );
}
else { // if not, load these styles
add_editor_style( get_site_url() . $standard_post_css . '?v=' . filemtime( ABSPATH . $standard_post_css ) );
}
}
// If no post type is set, load these styles for new a "post"
else {
add_editor_style( get_site_url() . $standard_post_css . '?v=' . filemtime( ABSPATH . $standard_post_css ) );
}
}
// Styles that get loaded when editing a post
if ( stristr( $_SERVER['REQUEST_URI'], 'post.php' ) !== false
&& is_object( $post ) ) {
if ( in_array( get_post_type( $post->ID ) , $my_post_types) // if in our specified post types
|| has_term( $my_tax_terms , $my_tax , $post->ID) // or has our specified taxonomy terms
|| has_term( $my_tags , 'post_tag', $post->ID) ) { // or has our specified tags
add_editor_style( get_site_url() . $blank_canvas_css . '?v=' . filemtime( get_home_path() . $blank_canvas_css ) );
}
else {
add_editor_style( get_site_url() . $standard_post_css . '?v=' . filemtime( get_home_path() . $standard_post_css ) );
}
}
}
add_theme_support( 'editor-styles' );
add_action( 'pre_get_posts', 'newpulse_add_editor_styles' );
Take a minute to go through each line and see if you’re able to get a general understanding of what’s going on.
You’ll want to of course replace the example conditions and stylesheets with your own.
More on that below.
Understanding The Snippet
The snippet is meant to serve as a buffet of options, and you’ll likely want to cut some parts out.
You probably don’t need a combination of all the conditions listed in the snippet.
Setting your post types, taxonomy terms, and tags
We establish what post types we want to use in our condition by adding them to an array contained in a variable:
$my_post_types = array("digital-product", "another-post-type");
You can create more than one variable if you want to use different sets of post types for different conditions.
Setting your stylesheets
We include our external stylesheet location in a variable as well.
$standard_post_css = "https://newpulselabs.b-cdn.net/wp-content/uploads/gutenberg-post-styling.css";
That way we if we ever need to change the URL in the future, we only need to make the change in one place.
Styles loaded when creating a new post vs. editing an existing post
Because the page URL is different when first creating a post vs. editing an existing post, we need two sections to specify our conditions for each.
However, we can’t apply as many conditions to new posts because if we’re creating a brand new post, we haven’t had a chance to add tags or taxonomies yet.
Therefor for new posts it only makes sense to apply conditions based on post type.
If you’re creating conditions based on taxonomy terms or tags, do it in the Editing Post section.
Bonus: Cache busting
You might wondering what this part of the snippet is for:
'?v=' . filemtime( ABSPATH . $standard_post_css )
It adds the file modified time as a URL parameter to the end of your file name.
That way whenever you modify a CSS file, your browser will retrieve the latest version.
Without it, your browser, CDN, or Cloudflare may cache the initial version of your CSS file and you won’t see any of your recent changes inside of Gutenberg.
Additional Note: for new posts we use ABSPATH, and for editing posts we use get_site_url().
This is because get_site_url() isn’t yet available to us when first creating a post.
A Few Examples
Because the snippet above contains a variety of different conditions, it might look a little overwhelming if you’re just trying to load a stylesheet based on a simple condition.
Here are a few examples of what your snippet might look like if just using one type of condition.
Load Gutenberg styling based on post types
<?php
/**
* Registers Gutenberg editor stylesheets based on custom post types.
*
* @global WP_Post $post Global post object.
*/
function newpulse_add_editor_styles() {
global $post;
$my_post_types = array("digital-product", "another-post-type"); // replace with your CPT slugs
$standard_post_css = "https://newpulselabs.b-cdn.net/wp-content/uploads/gutenberg-post-styling.css"; // example stylesheet for blog posts
$blank_canvas_css = "/wp-content/uploads/gutenberg-blank-canvas-styling.css"; // example stylesheet for full pages
// Styles that get loaded when first creating a new post
if ( false !== stristr( $_SERVER['REQUEST_URI'], 'post-new.php' ) ) {
if ( isset( $_GET['post_type'] ) === true ) { // checks if there is a custom post type
if ( in_array( $_GET['post_type'] , $my_post_types) ) { // if in our specified post types, load these styles
add_editor_style( get_site_url() . $blank_canvas_css . '?v=' . filemtime( ABSPATH . $blank_canvas_css ) );
}
else { // if not, load these styles
add_editor_style( get_site_url() . $standard_post_css . '?v=' . filemtime( ABSPATH . $standard_post_css ) );
}
}
// If no post type is set, load these styles for new a "post"
else {
add_editor_style( get_site_url() . $standard_post_css . '?v=' . filemtime( ABSPATH . $standard_post_css ) );
}
}
// Styles that get loaded when editing a post
if ( stristr( $_SERVER['REQUEST_URI'], 'post.php' ) !== false
&& is_object( $post ) ) {
if ( in_array( get_post_type( $post->ID ) , $my_post_types) // if in our specified post types
) {
add_editor_style( get_site_url() . $blank_canvas_css . '?v=' . filemtime( get_home_path() . $blank_canvas_css ) );
}
else {
add_editor_style( get_site_url() . $standard_post_css . '?v=' . filemtime( get_home_path() . $standard_post_css ) );
}
}
}
add_theme_support( 'editor-styles' );
add_action( 'pre_get_posts', 'newpulse_add_editor_styles' );
Load Gutenberg styling based on taxonomy terms
<?php
/**
* Registers Gutenberg editor stylesheets based on taxonomy terms.
*
* @global WP_Post $post Global post object.
*/
function newpulse_add_editor_styles() {
global $post;
$my_tax = 'digital_product_page_type'; // replace with your taxonomy slug
$my_tax_terms = array("landing-page"); // replace with taxonomy term slugs
$standard_post_css = "https://newpulselabs.b-cdn.net/wp-content/uploads/gutenberg-post-styling.css"; // example stylesheet for blog posts
$blank_canvas_css = "/wp-content/uploads/gutenberg-blank-canvas-styling.css"; // example stylesheet for full pages
// Styles that get loaded when editing a post
if ( stristr( $_SERVER['REQUEST_URI'], 'post.php' ) !== false
&& is_object( $post ) ) {
if ( has_term( $my_tax_terms , $my_tax , $post->ID) // if has our specified taxonomy terms
) {
add_editor_style( get_site_url() . $blank_canvas_css . '?v=' . filemtime( get_home_path() . $blank_canvas_css ) );
}
else {
add_editor_style( get_site_url() . $standard_post_css . '?v=' . filemtime( get_home_path() . $standard_post_css ) );
}
}
}
add_theme_support( 'editor-styles' );
add_action( 'pre_get_posts', 'newpulse_add_editor_styles' );
Load Gutenberg styling based on post tags
<?php
/**
* Registers Gutenberg editor stylesheets based on post tags.
*
* @global WP_Post $post Global post object.
*/
function newpulse_add_editor_styles() {
global $post;
$my_tags = array("gutenberg-full"); // replace with your tag slugs
$standard_post_css = "https://newpulselabs.b-cdn.net/wp-content/uploads/gutenberg-post-styling.css"; // example stylesheet for blog posts
$blank_canvas_css = "/wp-content/uploads/gutenberg-blank-canvas-styling.css"; // example stylesheet for full pages
// Styles that get loaded when editing a post
if ( stristr( $_SERVER['REQUEST_URI'], 'post.php' ) !== false
&& is_object( $post ) ) {
if ( has_term( $my_tags , 'post_tag', $post->ID) ) { // if has our specified tags
add_editor_style( get_site_url() . $blank_canvas_css . '?v=' . filemtime( get_home_path() . $blank_canvas_css ) );
}
else {
add_editor_style( get_site_url() . $standard_post_css . '?v=' . filemtime( get_home_path() . $standard_post_css ) );
}
}
}
add_theme_support( 'editor-styles' );
add_action( 'pre_get_posts', 'newpulse_add_editor_styles' );
Final Thoughts
Hopefully you found these snippets useful.
Depending on your situation, they should make Gutenberg a lot easier to use and closer resemble the front-end of your site.
Note: I don’t consider myself a PHP expert, so if you have any improvements to make to the snippet, feel free to leave a comment below 🙂